Java Full Stack
learn Java full stack from industry experts.
Earn a recognized certification to boost your career.
Work on real world projects
Master skills java, JavaScript,Html,CSS and more.
Overview
Embark on a journey to become a Java Full Stack Developer with our in-depth course. This program covers both front-end and back-end technologies, focusing on Java, Spring Boot for the backend, and React or Angular for the frontend. You’ll also learn about databases, API development, and deployment, equipping you with the skills to build robust, scalable applications.
Key Highlights
- Comprehensive Curriculum: From Java fundamentals to advanced web development.
- Hands-On Projects: Apply your skills by building real-world applications.
- Career Readiness: Prepare for a career in software development with targeted training and support.
Who Can Apply?
- Individuals new to programming and web development.
- Experienced developers looking to specialize in Java full stack.
- Students and professionals seeking a career transition into tech.
Curriculum
Core Java
Introduction To Java
- History And Features Of Java
- Installation Of Java Development Kit (Jdk)
- Setting Up The Development Environment
Basic Syntax And Data Types
- Variables And Data Types (Primitive And Reference Types)
- Operators And Expressions
- Control Flow Statements (If, Else, Switch, Loops)
Object-Oriented Programming (Oop)
- Classes And Objects
- Constructors And Methods
- Inheritance, Polymorphism, And Encapsulation
- Abstract Classes And Interfaces
Exception Handling
-
Understanding Exceptions Try-Catch Blocks
- Handling Exceptions Using Throws And Throw Keywords
- Custom Exceptions
Collections Framework
- Overview Of Collections Framework
- Lists, Sets, Maps
- Iterators And Foreach Loop
- Working With Array List, Linkedlist, Hashset, Hashmap, Etc.
File Handling
- Reading From And Writing To Files
- File Input/Output Streams
- Exception Handling In File Operations
Multithreading
- Introduction To Threads
- Creating And Running Threads
- Synchronization And Thread Safety ·
- Thread Communication And Coordination
Input/Output (I/O)
- Streams And Readers/Writers
- File I/O Using Fileinputstream, Fileoutputstream, Etc.
- Serialization And Deserialization
Generics
- Introduction To Generics ·
- Generic Classes And Methods · ·
- Wildcards And Bounded Types
Lambda Expressions And Functional Interfaces
- Introduction To Functional Programming Concepts
- Defining And Using Lambda Expressions
- Built-In Functional Interfaces In Java 8 (E.G., Predicate, Function, Consumer)
Advanced Java
Advanced Oop Concepts
- Design Patterns (E.G., Singleton, Factory, Observer, Strategy)
- Composition Vs. Inheritance ·
- Polymorphism In Depth (Dynamic Method Dispatch, Overriding Vs. Overloading) ·
- Object Cloning And Serialization
Java Collections Framework
- Concurrent Collections (E.G., Concurrenthashmap, Copyonwritearraylist) ·
- Custom Collections (Implementing Iterable, Comparator, Etc.) ·
- Collections Utility Methods (E.G., Sorting, Searching, Filtering)
Concurrency And Multithreading
- Advanced Threading Concepts (E.G., Thread Pools, Executors)
- Concurrent Utilities (E.G., Semaphore, Countdown Latch, Cyclic Barrier)
- Thread Synchronization Mechanisms (E.G., Volatile Keyword, Synchronized Blocks/Methods)
Networking And I/O
- Socket Programming (Client-Server Communication)
- Non-Blocking I/O (Nio) And Java. Nio Package
- Asynchronous I/O (Java Nio.2)
Database Connectivity
- Jdbc (Java Database Connectivity) Api
- Connection Pooling And Datasource
- Transactions And Isolation Levels
Annotations And Reflection
- Annotation Processing And Custom Annotations
- Reflection Api (Introspection, Obtaining Class Information At Runtime)
- Annotation-Based Frameworks (E.G., Spring Framework)
Java Ee (Enterprise Edition) Technologies
- Servlets And Jsp (Java Server Pages)
- Java Server Faces (Jsf)
- Enterprise Javabeans (Ejb)
- Java Persistence Api (Jpa) And Orm Frameworks (E.G., Hibernate)
Web Services
-
Introduction To Soap And Restful Web Services
-
Implementing Web Services Using Jax-Ws And Jax-Rs
-
Consuming Web Services In Java Applications
Spring Framework
- Dependency Injection (Di) And Inversion Of Control (Ioc)
- Spring Core Modules (Spring Core, Spring Mvc, Spring Boot)
- Spring Data, Spring Security, And Other Spring Modules
HTML & CSS
Introduction To Web Development
- Overview Of Web Technologies
- Understanding Client-Side Vs. Server-Side Development
- Introduction To Html, Css, And Their Roles In Web Development
Html Fundamentals
- Understanding The Structure Of Html Documents (Html5 Doctype)
- Html Elements, Tags, And Attributes
- Text Formatting (Headings, Paragraphs, Lists, Links)
- Semantic Html (Div Vs. Semantic Elements Like Header, Nav, Section, Article, Footer)
- Html Forms And Form Elements
CSS Fundamentals
Introduction To Css And Its Role In Styling Web
- Pages Css Syntax (Selectors, Properties, Values)
- Internal Vs. External Vs. Inline Css
- Cascading And Inheritance
- Box Model (Margin, Border, Padding, Width, Height)
- Css Units (Pixels, Percentages, Ems, Rems)
Styling With Css
- Text Styling (Font Properties, Text Alignment, Text Decoration)
- Colors And Backgrounds
- Layout Techniques (Floats, Positioning, Flexbox, Grid)
- Responsive Web Design Principles
- Media Queries And Viewport Meta Tag
Css Selectors And Pseudo-Classes
- Understanding Css Selectors (Element Selectors, Class Selectors, Id Selectors, Descendant Selectors)
- Pseudo-Classes (Hover, Active, Focus, Nth-Child, Etc.) Specificity And The Importance Of Selector Precedence
Css Layout Techniques
- Float-Based Layout
- Css Positioning (Static, Relative, Absolute, Fixed)
- Flexbox Layout Model
- Css Grid Layout
Advanced Css Features
- Css Transitions And Animations
- Css Preprocessors (E.G., Sass, Less) And Their Advantages
- Css Frameworks (E.G., Bootstrap) And Their Usage
Accessibility And Best Practices
- Writing Accessible Html Markup (Semantic Elements, Alt Attributes, Aria Roles)
- Best Practices For Writing Clean And Maintainable Html And Css Code Performance Optimization Techniques
Project Work
- Implementing Real-World Projects To Apply Learned Concepts
- Developing Static Web Pages And Simple Websites Using Html And Css
- Incorporating Responsive Design Principles Into Projects
Java Script
Introduction To Javascript
- History And Evolution Of Javascript
- Role Of Javascript In Web Development
- Setting Up A Javascript Development Environment
Basic Javascript Concepts
- Variables, Data Types, And Operators
- Control Flow Statements (If, Else, Switch)
- Loops (For, While, Do-While)
- Functions (Declaration, Expressions, Parameters, Return Values)
Working With Arrays And Objects
- Creating And Manipulating Arrays
- Iterating Through Arrays Using Loops And Array Methods
- Understanding Object-Oriented Programming Concepts In Javascript
- Creating And Working With Objects And Their Properties/Methods
Dom Manipulation
- Introduction To The Document Object Model (Dom)
- Accessing And Modifying Html Elements Using Javascript
- Event Handling (Click, Hover, Submit, Etc.)
- Dom Traversal And Manipulation Techniques
Asynchronous Javascript
- Understanding Asynchronous Programming
- Callback Functions
- Promises And Asynchronous Functions (Async/Await)
- Handling Asynchronous Operations (Ajax Requests, Set Timeout, Set Interval)
Error Handling
- Understanding Javascript Errors And Exceptions
- Using Try-Catch Blocks For Error Handling
- Throwing And Catching Custom Errors
Functional Programming Concepts
- Introduction To Functional Programming In Javascript
- Higher-Order Functions
- Array Methods (Map, Filter, Reduce)
- Arrow Functions And Their Advantages
ES6+ Features
- Overview Of Ecmascript 6 (ES6) Features And Syntax Enhancements Let And Const Variables
- Arrow Functions, Template Literals, And Default Parameters
- Destructuring, Rest Parameters, And Spread Syntax
Browser Apis And Events
- Introduction To Browser Apis (Local Storage, Sessionstorage, Fetch Api, Etc.)
- Working With Browser Events
- Creating Interactive Web Pages Using Javascript And Browser Apis
Introduction To Modern Javascript Frameworks (Optional)
- Overview Of Popular Javascript Frameworks/Libraries (E.G., React.Js, Angular, Vue.Js)
- Understanding The Role Of Frameworks In Modern Web Development
SQL
Introduction
- What Is Data
- Types Of Data
- What Is Database
- Types Of Databases
- What Is A Table
Operations On Table
- Creation Of Table
- Select Statement
Data Modifications
- Insert Data Into Tables
- Insert Statements & Insert Into Select Statements
- Updating Existing Data
- Deleting Data From Table
- Drop
- Truncating Tables
- Alter Statements
Constraints
- Primary Key
- Foreign Key
- Unique Key
- Not Null
- Check
- Default
Indexes
- Understanding Indexes And Their Importance
- Creating And Dropping Indexes
- Index (B Tree, Hash)
Views
- Creating Views
- Modifying Views
- Dropping Views
- Updating Data Through View
Data Retrieval
- Retrieving Data From A Single Table
- Where Clause
- Group By Clause
- Having Clause
- Order By Clause
- Limit Clause
- Case Statement
- Case Statement For Validating The Data Based On Condition
Joins
- Inner Join
- Left Join
- Right Join
- Full Join
- Cross Join
- Self-Join
Functions
- Numerical Functions
- Date Functions
- String Functions
- Aggregate Functions
Set Operator
- Union
- Intersect
- Minus
- Except
- Union All
Sub Ǫueries
- Single Row
- Multiple Row
- Scalar Row
- Correlated
- Exist
- Not Exist
- From And Select
- Where And From
Stored Procedure
- Create
- Dml
- Tcl (Commit,Roll Back, Savepoints,Acid)
- Cursor
- Execution
- Passing Parameters To Stored Procedures And Functions
- Invoking Stored Procedures And Functions
Advance Sǫl Topics
- Windows Functions
- Common Table Expression
- Recursion
- Pivot And Unpivot Operation
- Dynamic Sǫl
Corporate Training
We give Corporate Employees the Training They Need to Learn & Lead
Details
Flexible Timings
36 Hours Training
Certification
24/7 Support
Courses focused on building strong foundational skills for career growth
- Versatility: Become proficient in both front-end and back-end technologies, enabling you to independently develop comprehensive web applications.
- Career Opportunities: Full-stack developers are highly sought after across industries, providing ample career advancement opportunities and job stability.
- Hands-On Experience: Work on real-world projects to gain practical skills in application development, preparing you for challenges in professional settings.
- Relevance: Java, Spring, and Hibernate are widely used in enterprise-level web development, ensuring that skills acquired are aligned with current industry practices.
- Comprehensive Skill Set: Master client-server programming, database management, and deployment techniques, equipping yourself with essential skills for modern web development projects.
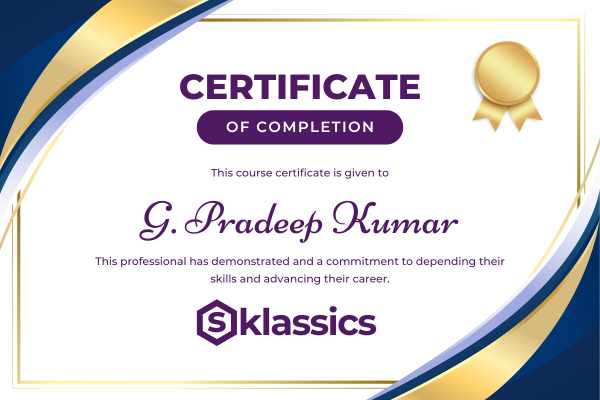
Student Success Stories
Frequently Asked Questions
What frameworks and technologies are covered in the Java Full Stack course?
The course covers Java programming, along with front-end technologies like HTML, CSS, JavaScript, and back-end frameworks such as Spring and Hibernate.
How will real project experience benefit me in this course?
Real project experience allows you to apply theoretical knowledge in practical scenarios, helping you understand the complexities of full-stack development and preparing you for real-world challenges.
Are there any prerequisites for enrolling in the Java Full Stack course?
Basic understanding of programming concepts and familiarity with Java would be beneficial. Some exposure to web technologies like HTML, CSS, and JavaScript is also recommended.
What career opportunities can a Java Full Stack certification open up?
A Java Full Stack certification demonstrates your proficiency in building complete web applications using Java and related technologies, making you eligible for roles such as full-stack developer, Java developer, or web application developer in various industries.
Related Courses
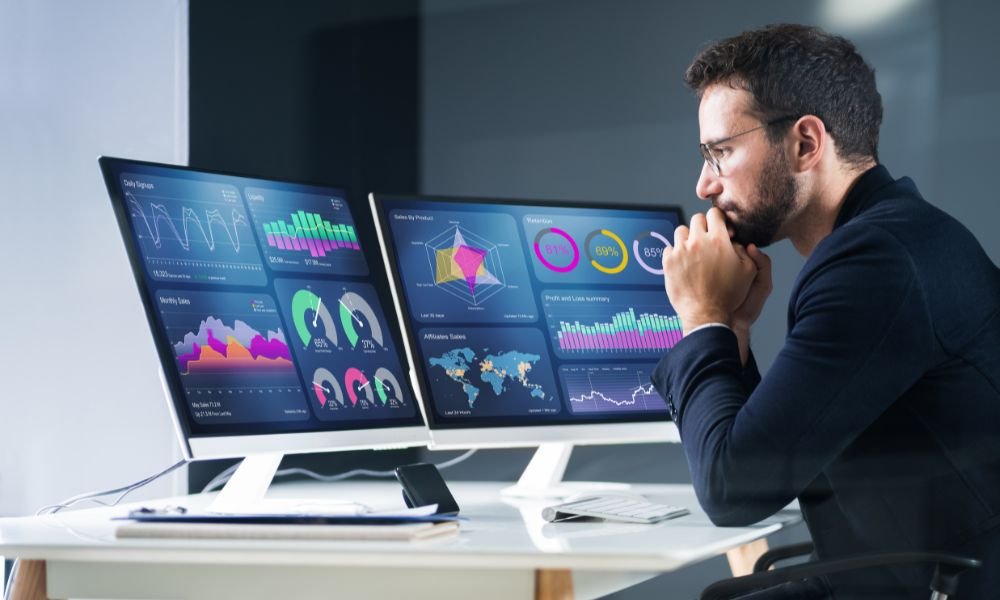
Business Analytics
Buy for ₹20,000
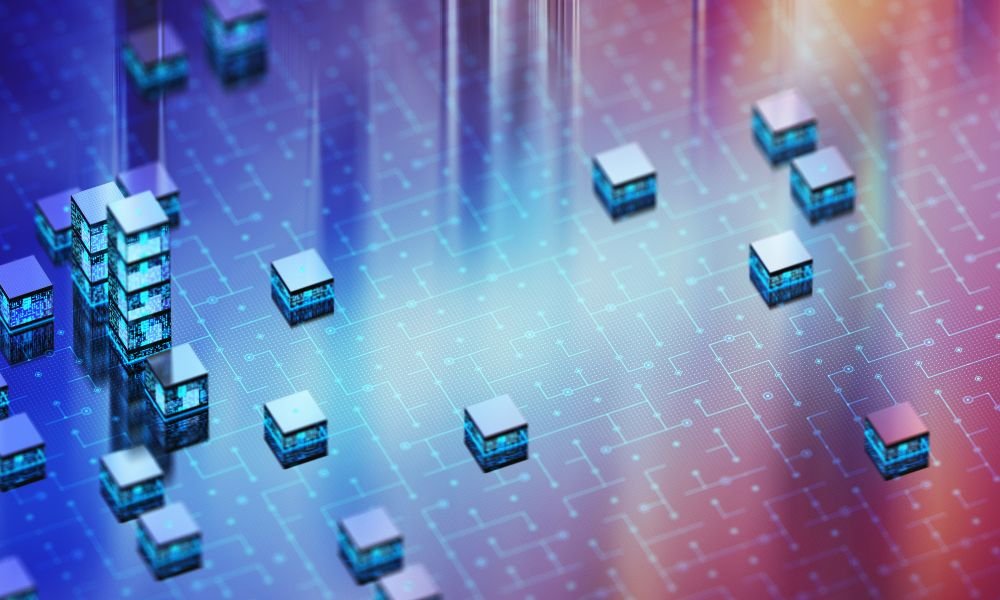
Big Data Analytics
Buy for ₹30,000
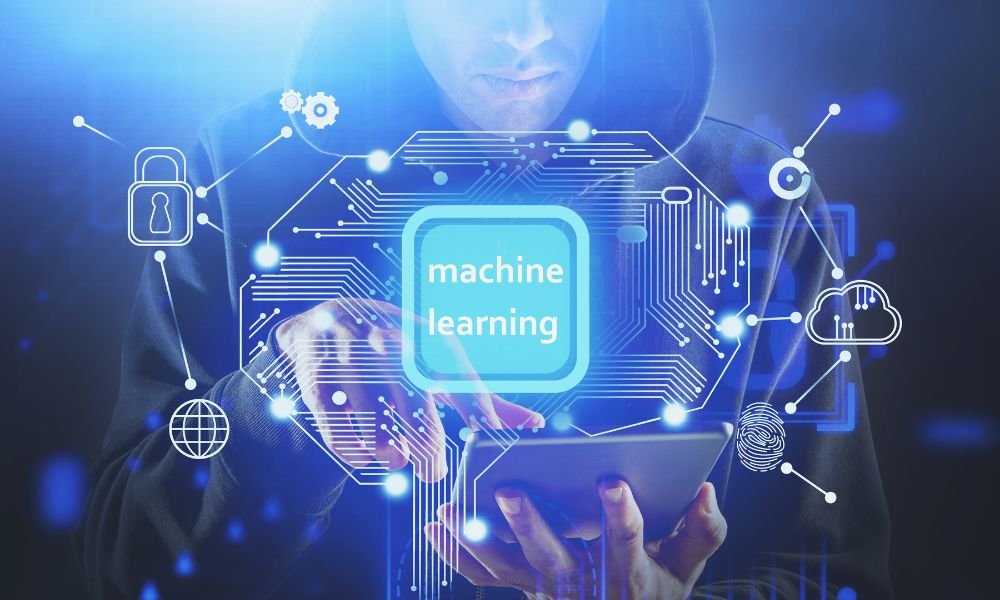
Machine Learning
Buy for ₹20,000
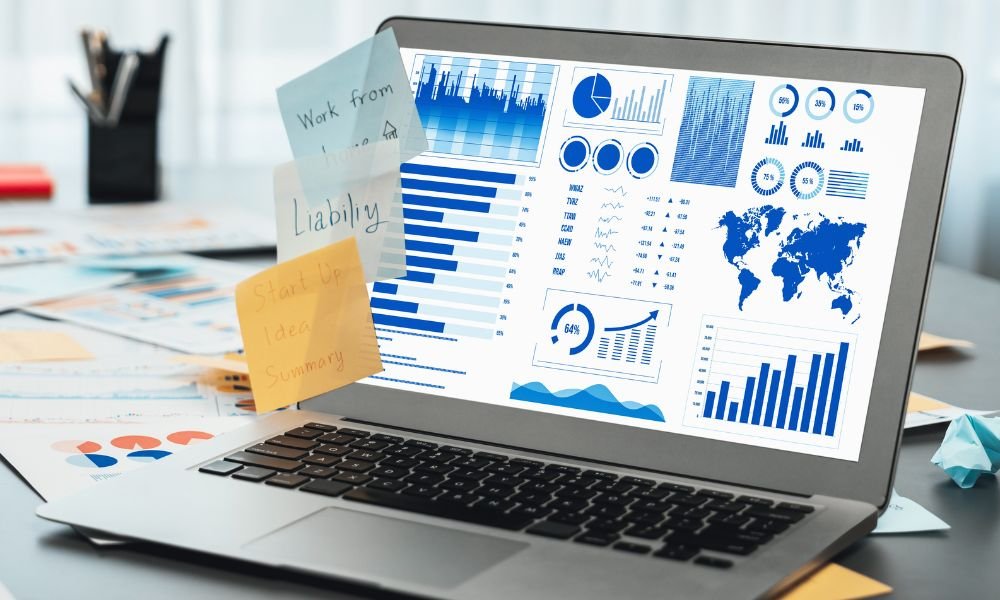
Power BI
Buy for ₹15,000